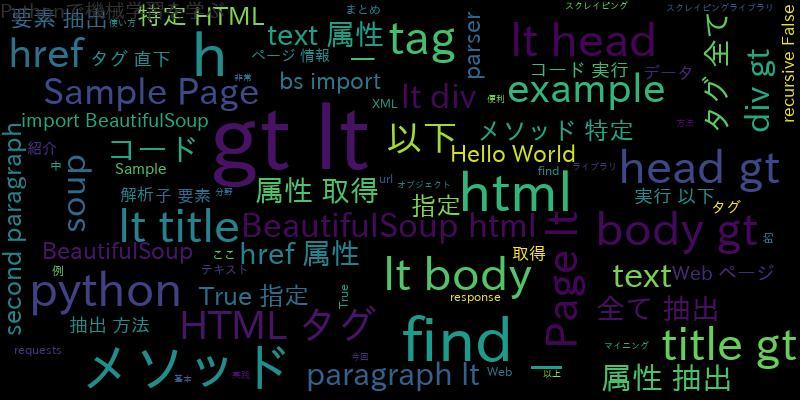
PythonのWebスクレイピングライブラリであるBeautifulSoupは、HTMLやXMLの解析によく使われます。その中でも、find_all
メソッドは、特定のHTMLタグを全て抽出することができる非常に便利なメソッドです。
BeautifulSoupとは?
BeautifulSoupは、HTMLやXMLなどのテキストデータを解析するためのPythonライブラリです。Webスクレイピングやデータマイニングなどの分野でよく使われます。BeautifulSoupは、解析したテキストデータをPythonのオブジェクトとして扱うことができます。そのため、Pythonのコードで解析したデータを処理することができます。
BeautifulSoupのfind_allメソッドの基本的な使い方
BeautifulSoupのfind_all
メソッドは、特定のHTMLタグを全て抽出することができます。例えば、以下のようなHTMLコードがあったとします。
<html> <head> <title>Sample Page</title> </head> <body> <h1>Hello World!</h1> <p>This is a sample page.</p> <p>This is the second paragraph.</p> </body> </html>
このHTMLコードから、<p>
タグを全て抽出するには、以下のようにfind_all
メソッドを使います。
from bs4 import BeautifulSoup html = """ <html> <head> <title>Sample Page</title> </head> <body> <h1>Hello World!</h1> <p>This is a sample page.</p> <p>This is the second paragraph.</p> </body> </html> """ soup = BeautifulSoup(html, "html.parser") p_tags = soup.find_all("p") print(p_tags)
このコードを実行すると、以下のように<p>
タグが全て抽出されます。
[<p>This is a sample page.</p>, <p>This is the second paragraph.</p>]
このように、find_all
メソッドは、特定のHTMLタグを全て抽出することができます。
find_allメソッドで子要素を抽出する方法
find_all
メソッドは、指定したHTMLタグの直下にある子要素だけを抽出することもできます。例えば、以下のようなHTMLコードがあったとします。
<html> <head> <title>Sample Page</title> </head> <body> <div> <p>This is a sample paragraph.</p> </div> <div> <p>This is the second paragraph.</p> </div> </body> </html>
このHTMLコードから、<div>
タグの直下にある<p>
タグを全て抽出するには、以下のようにfind_all
メソッドにrecursive=False
を指定します。
from bs4 import BeautifulSoup html = """ <html> <head> <title>Sample Page</title> </head> <body> <div> <p>This is a sample paragraph.</p> </div> <div> <p>This is the second paragraph.</p> </div> </body> </html> """ soup = BeautifulSoup(html, "html.parser") p_tags = soup.find_all("p", recursive=False) print(p_tags)
このコードを実行すると、以下のように<div>
タグの直下にある<p>
タグが全て抽出されます。
[<p>This is a sample paragraph.</p>, <p>This is the second paragraph.</p>]
このように、find_all
メソッドにrecursive=False
を指定することで、指定したHTMLタグの直下にある子要素だけを抽出することができます。
find_allメソッドでhref属性を抽出する方法
find_all
メソッドを使って、a
タグのhref
属性を抽出することもできます。例えば、以下のようなHTMLコードがあったとします。
<html> <head> <title>Sample Page</title> </head> <body> <a href="http://example.com">Example</a> </body> </html>
このHTMLコードから、<a>
タグのhref
属性を抽出するには、以下のようにfind_all
メソッドにhref=True
を指定します。
from bs4 import BeautifulSoup html = """ <html> <head> <title>Sample Page</title> </head> <body> <a href="http://example.com">Example</a> </body> </html> """ soup = BeautifulSoup(html, "html.parser") a_tags = soup.find_all("a", href=True) for a_tag in a_tags: print(a_tag["href"])
このコードを実行すると、以下のように<a>
タグのhref
属性が抽出されます。
http://example.com
このように、find_all
メソッドにhref=True
を指定することで、a
タグのhref
属性を抽出することができます。
find_allメソッドでtext属性を取得する方法
find_all
メソッドを使って、HTMLタグのtext
属性を取得することもできます。例えば、以下のようなHTMLコードがあったとします。
<html> <head> <title>Sample Page</title> </head> <body> <h1>Hello World!</h1> </body> </html>
このHTMLコードから、<h1>
タグのtext
属性を取得するには、以下のようにfind_all
メソッドにtext=True
を指定します。
from bs4 import BeautifulSoup html = """ <html> <head> <title>Sample Page</title> </head> <body> <h1>Hello World!</h1> </body> </html> """ soup = BeautifulSoup(html, "html.parser") h1_tags = soup.find_all("h1", text=True) for h1_tag in h1_tags: print(h1_tag.text)
このコードを実行すると、以下のように<h1>
タグのtext
属性が取得されます。
Hello World!
このように、find_all
メソッドにtext=True
を指定することで、HTMLタグのtext
属性を取得することができます。
BeautifulSoupのfind_allメソッドを使った実践的な例
ここまでで、find_all
メソッドを使って、特定のHTMLタグや属性を抽出する方法を学びました。ここでは、実際にWebページから情報を取得する例を紹介します。
例えば、以下のようなWebページがあったとします。
<html> <head> <title>Example Page</title> </head> <body> <h1>Example Page</h1> <ul> <li> <a href="http://example.com/page1">Page 1</a> </li> <li> <a href="http://example.com/page2">Page 2</a> </li> <li> <a href="http://example.com/page3">Page 3</a> </li> </ul> </body> </html>
このWebページから、<ul>
タグの中にある<a>
タグのhref
属性とtext
属性を取得するには、以下のようにfind_all
メソッドを使います。
from bs4 import BeautifulSoup import requests url = "http://example.com" response = requests.get(url) soup = BeautifulSoup(response.text, "html.parser") ul_tag = soup.find("ul") for li_tag in ul_tag.find_all("li"): a_tag = li_tag.find("a") href = a_tag["href"] text = a_tag.text print(href, text)
このコードを実行すると、以下のように<a>
タグのhref
属性とtext
属性が取得されます。
http://example.com/page1 Page 1 http://example.com/page2 Page 2 http://example.com/page3 Page 3
このように、find_all
メソッドを使って、Webページから情報を取得することができます。
まとめ
今回は、BeautifulSoupのfind_all
メソッドを使って、特定のHTMLタグや属性を抽出する方法を紹介しました。以下に、まとめを記します。
find_all
メソッドは、特定のHTMLタグを全て抽出することができる。find_all
メソッドにrecursive=False
を指定することで、指定したHTMLタグの直下にある子要素だけを抽出することができる。find_all
メソッドにhref=True
を指定することで、a
タグのhref
属性を抽出することができる。find_all
メソッドにtext=True
を指定することで、HTMLタグのtext
属性を取得することができる。find_all
メソッドを使って、Webページから情報を取得することができる。
以上が、BeautifulSoupのfind_all
メソッドを使って、HTMLタグや属性を抽出する方法についての紹介でした。