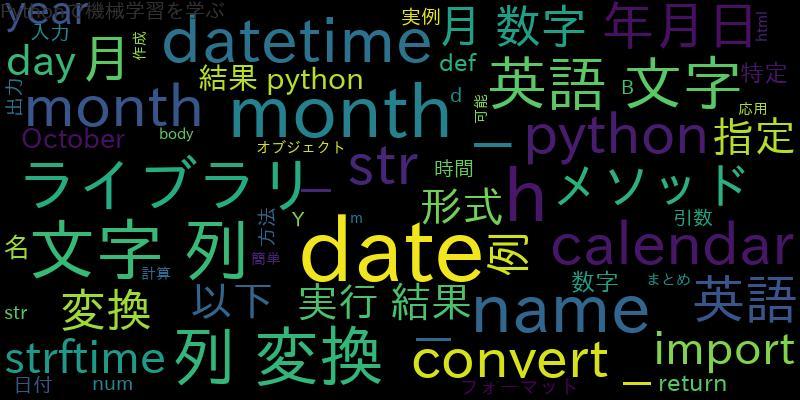
Pythonのdatetimeライブラリについて
Pythonには、日付や時間を扱うためのdatetimeライブラリがあります。このライブラリを使うことで、日付や時間の計算やフォーマットなどが簡単に行えます。
年月日を英語の文字列に変換する方法
年月日を英語の文字列に変換するには、datetimeライブラリのstrftimeメソッドを使います。以下は、datetimeオブジェクトを作成し、strftimeメソッドで英語の文字列に変換する例です。
import datetime date = datetime.date(2023, 10, 25) date_str = date.strftime('%B %d, %Y') print(date_str)
実行結果:
October 25, 2023
strftimeメソッドの引数には、変換したい形式を指定します。%Bは、英語の月名を表すフォーマット文字列です。
月を英語の文字列に変換する方法
月を英語の文字列に変換するには、calendarライブラリのmonth_nameメソッドを使います。以下は、月の数字を指定し、month_nameメソッドで英語の文字列に変換する例です。
import calendar month_num = 10 month_name = calendar.month_name[month_num] print(month_name)
実行結果:
October
month_nameメソッドは、引数に月の数字を指定すると、その月の英語の月名を返します。
年月日を数字から英語に変換する実例
以下は、年月日の数字を入力として受け取り、英語の文字列に変換する例です。
import datetime import calendar def convert_date(year, month, day): date = datetime.date(year, month, day) month_name = calendar.month_name[month] date_str = month_name + ' ' + str(day) + ', ' + str(year) return date_str date_str = convert_date(2023, 10, 25) print(date_str)
実行結果:
October 25, 2023
convert_date関数では、datetimeライブラリとcalendarライブラリを使って、年月日を英語の文字列に変換しています。
月を数字から英語に変換する実例
以下は、月の数字を入力として受け取り、英語の月名に変換する例です。
import calendar def convert_month(month): month_name = calendar.month_name[month] return month_name month_name = convert_month(10) print(month_name)
実行結果:
October
convert_month関数では、calendarライブラリを使って、月の数字を英語の月名に変換しています。
応用例:特定の形式での出力
以下は、年月日を指定した特定の形式の文字列に変換する例です。
import datetime def convert_date(year, month, day): date = datetime.date(year, month, day) date_str = date.strftime('%Y-%m-%d') return date_str date_str = convert_date(2023, 10, 25) print(date_str)
実行結果:
2023-10-25
convert_date関数では、strftimeメソッドを使って、指定した形式の文字列に変換しています。
まとめ
Pythonのdatetimeライブラリとcalendarライブラリを使うことで、年月日を英語の文字列に変換することができます。また、月を数字から英語に変換することもできます。特定の形式での出力も可能です。