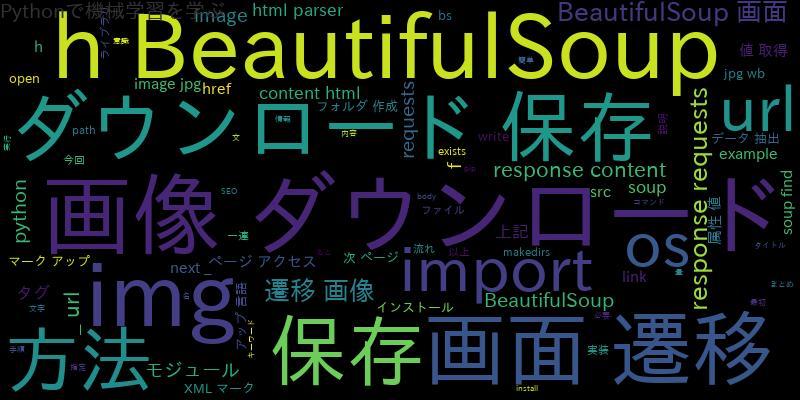
今回は、BeautifulSoupを使って画面遷移から画像をダウンロードし保存する方法について説明します。
BeautifulSoupとは?
BeautifulSoupは、HTMLやXMLなどのマークアップ言語からデータを抽出するためのPythonライブラリです。HTMLやXMLなどのマークアップ言語において、タグの情報をもとにデータを抽出することができます。
BeautifulSoupのインストール方法
BeautifulSoupを使うには、まずはインストールが必要です。
pip install beautifulsoup4
上記のコマンドを実行することで、BeautifulSoupをインストールすることができます。
BeautifulSoupでの画面遷移の方法
BeautifulSoupを使って画面遷移をするには、requests
モジュールを使います。
import requests from bs4 import BeautifulSoup url = "http://example.com" response = requests.get(url) soup = BeautifulSoup(response.content, "html.parser") # aタグを探して、href属性の値を取得する link = soup.find("a") next_url = link.get("href") # 次のページにアクセスする response = requests.get(next_url) soup = BeautifulSoup(response.content, "html.parser")
上記のように、requests
モジュールを使って次のページにアクセスすることができます。
BeautifulSoupでの画像ダウンロードの方法
BeautifulSoupを使って画像をダウンロードするには、requests
モジュールを使います。
import requests url = "http://example.com/image.jpg" response = requests.get(url) # ファイル名を指定して保存する with open("image.jpg", "wb") as f: f.write(response.content)
上記のように、requests
モジュールを使って画像をダウンロードし、ファイルに保存することができます。
BeautifulSoupでの画像保存の方法
BeautifulSoupを使って画像を保存するには、os
モジュールを使います。
import os from bs4 import BeautifulSoup url = "http://example.com" response = requests.get(url) soup = BeautifulSoup(response.content, "html.parser") # imgタグを探して、src属性の値を取得する img = soup.find("img") img_url = img.get("src") # 画像を保存するフォルダを作成する if not os.path.exists("images"): os.makedirs("images") # 画像を保存する response = requests.get(img_url) with open("images/image.jpg", "wb") as f: f.write(response.content)
上記のように、os
モジュールを使って画像を保存するフォルダを作成し、画像を保存することができます。
BeautifulSoupを使った画面遷移から画像ダウンロード、保存までの一連の流れ
以上の手順を組み合わせることで、画面遷移から画像をダウンロードし保存することができます。
import requests import os from bs4 import BeautifulSoup # 最初のページにアクセスする url = "http://example.com" response = requests.get(url) soup = BeautifulSoup(response.content, "html.parser") # aタグを探して、href属性の値を取得する link = soup.find("a") next_url = link.get("href") # 画像を保存するフォルダを作成する if not os.path.exists("images"): os.makedirs("images") # 次のページにアクセスする response = requests.get(next_url) soup = BeautifulSoup(response.content, "html.parser") # imgタグを探して、src属性の値を取得する img = soup.find("img") img_url = img.get("src") # 画像をダウンロードし、保存する response = requests.get(img_url) with open("images/image.jpg", "wb") as f: f.write(response.content)
上記のように、BeautifulSoupを使った画面遷移から画像ダウンロード、保存までの一連の流れを実装することができます。
まとめ
今回は、BeautifulSoupを使って画面遷移から画像をダウンロードし保存する方法について説明しました。BeautifulSoupは、HTMLやXMLなどのマークアップ言語からデータを抽出するためのPythonライブラリであり、画面遷移や画像ダウンロード、保存などの処理を簡単に実装することができます。