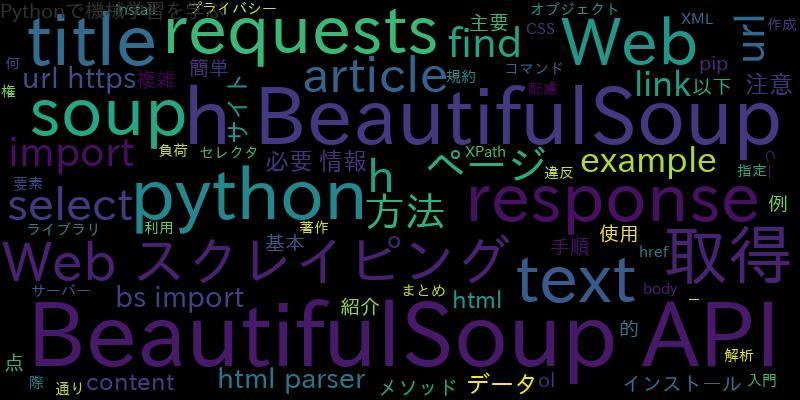
BeautifulSoup APIとは何か
BeautifulSoup APIは、PythonでWebスクレイピングを行うためのライブラリの一つです。WebページのHTMLやXMLを解析して、必要な情報を取得することができます。
BeautifulSoup APIのインストール方法
BeautifulSoup APIは、pipコマンドを使って簡単にインストールできます。
pip install beautifulsoup4
PythonとBeautifulSoup APIを使ったWebスクレイピングの基本的な手順
PythonとBeautifulSoup APIを使ってWebスクレイピングを行う基本的な手順は以下の通りです。
- WebページのHTMLを取得する
- BeautifulSoupオブジェクトを作成する
- 必要な情報を取得する
BeautifulSoup APIの主要なメソッドとその使用例
BeautifulSoup APIの主要なメソッドとその使用例を紹介します。
-
- find()
from bs4 import BeautifulSoup import requests url = 'https://www.example.com' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') title = soup.find('title') print(title.text)
-
- find_all()
from bs4 import BeautifulSoup import requests url = 'https://www.example.com' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') links = soup.find_all('a') for link in links: print(link.get('href'))
-
- select()
from bs4 import BeautifulSoup import requests url = 'https://www.example.com' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') title = soup.select('title') print(title[0].text)
BeautifulSoup APIを使って複雑なWebサイトからデータを取得する方法
BeautifulSoup APIを使って複雑なWebサイトからデータを取得する方法は、CSSセレクタやXPathを使って要素を指定することです。
from bs4 import BeautifulSoup import requests url = 'https://www.example.com' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') articles = soup.select('.article') for article in articles: title = article.select('.title')[0].text content = article.select('.content')[0].text print(title) print(content)
BeautifulSoup APIを使ったWebスクレイピングの注意点
Webスクレイピングを行う際には、以下の注意点に注意してください。
- Webページの利用規約に違反しないようにする
- Webサイトのサーバーに負荷をかけないようにする
- 取得したデータの著作権やプライバシーに配慮する
まとめ
BeautifulSoup APIを使ってWebスクレイピングを行う方法について紹介しました。PythonとBeautifulSoup APIを使えば、簡単にWebページから必要な情報を取得することができます。