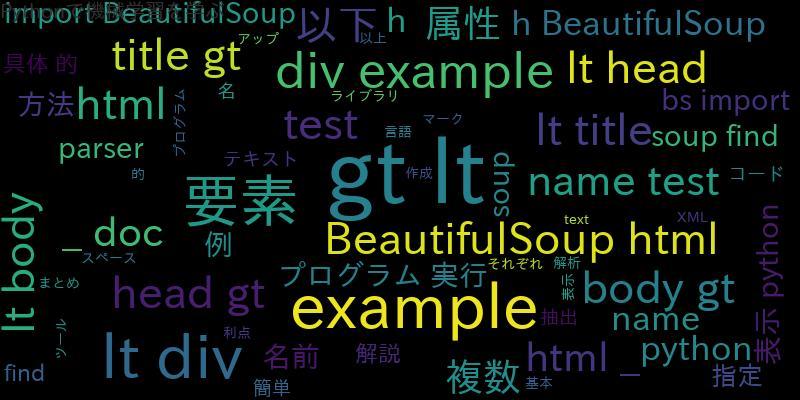
BeautifulSoupは、Pythonのライブラリであり、HTMLやXMLなどのマークアップ言語を解析するためのツールです。BeautifulSoupを使うと、HTMLの要素を簡単に抽出することができます。
BeautifulSoupでclassから要素を探す基本的な方法
HTMLの要素にはclass属性があります。class属性は、複数の要素に同じ名前を付けることができます。BeautifulSoupでは、class属性を指定して要素を探すことができます。
以下の例では、classが「example」の要素を探しています。
from bs4 import BeautifulSoup html_doc = """ <html> <head> <title>Example</title> </head> <body> <div class="example"> <p>This is an example.</p> </div> </body> </html> """ soup = BeautifulSoup(html_doc, 'html.parser') div = soup.find('div', {'class': 'example'}) print(div)
このプログラムを実行すると、以下のように「<div class=”example”>」の要素が表示されます。
<div class="example"> <p>This is an example.</p> </div>
BeautifulSoupでclassから複数のnameをfindする具体的な方法
class属性には、複数の名前を付けることもできます。その場合、スペースで区切って複数の名前を指定します。
以下の例では、classが「example」か「test」の要素を探しています。
from bs4 import BeautifulSoup html_doc = """ <html> <head> <title>Example</title> </head> <body> <div class="example test"> <p>This is an example.</p> </div> </body> </html> """ soup = BeautifulSoup(html_doc, 'html.parser') div = soup.find('div', {'class': ['example', 'test']}) print(div)
このプログラムを実行すると、以下のように「<div class=”example test”>」の要素が表示されます。
<div class="example test"> <p>This is an example.</p> </div>
複数のclass名を指定して要素を探す方法
class属性には、複数の名前を付けることができますが、それぞれの名前が付いた要素を探すこともできます。
以下の例では、classが「example」かつ「test」の要素を探しています。
from bs4 import BeautifulSoup html_doc = """ <html> <head> <title>Example</title> </head> <body> <div class="example test"> <p>This is an example.</p> </div> <div class="test"> <p>This is a test.</p> </div> </body> </html> """ soup = BeautifulSoup(html_doc, 'html.parser') div = soup.find('div', {'class': 'example test'}) print(div)
このプログラムを実行すると、以下のように「<div class=”example test”>」の要素が表示されます。
<div class="example test"> <p>This is an example.</p> </div>
まとめ
BeautifulSoupを使えば、HTMLの要素を簡単に抽出することができます。class属性とname属性を組み合わせて使うことで、より具体的な要素を探すことができます。複数のclass名を指定して要素を探すこともできます。
以上で、BeautifulSoupでclassから複数のnameをfindする方法を解説しました。