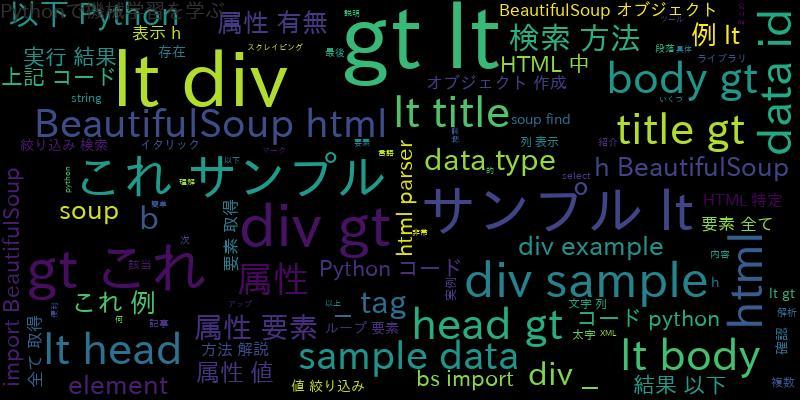
BeautifulSoupとは、HTMLやXMLなどのマークアップ言語を解析するためのPythonライブラリです。このライブラリを使えば、HTMLの特定の要素や属性を簡単に検索できます。この記事では、BeautifulSoupを使ったHTMLの属性の有無を検索する方法について解説します。
BeautifulSoupを使った属性の有無の確認方法
まずは、BeautifulSoupを使ってHTMLの特定の要素に属性があるかどうかを確認する方法を紹介します。以下のようなHTMLがあったとします。
<html> <head> <title>サンプル</title> </head> <body> <div class="sample">これはサンプルです</div> </body> </html>
このHTMLの中で、<div>要素にclass属性があるかどうかを確認するためには、以下のようなPythonコードを書きます。
from bs4 import BeautifulSoup html = """ <html> <head> <title>サンプル</title> </head> <body> <div class="sample">これはサンプルです</div> </body> </html> """ soup = BeautifulSoup(html, "html.parser") div_tag = soup.find("div", {"class": "sample"}) if div_tag: print("class属性があります") else: print("class属性はありません")
実行結果は以下のようになります。
class属性があります
上記のコードでは、BeautifulSoupオブジェクトを作成し、<div>要素を取得しています。そして、<div>要素が存在する場合には「class属性があります」と表示し、存在しない場合には「class属性はありません」と表示しています。
BeautifulSoupによるHTMLの特定の属性の検索方法
次に、BeautifulSoupを使ってHTMLの特定の属性を検索する方法について解説します。以下のようなHTMLがあったとします。
<html> <head> <title>サンプル</title> </head> <body> <div class="sample">これはサンプルです</div> <div class="sample">これもサンプルです</div> <div class="example">これは例です</div> </body> </html>
このHTMLの中で、class属性が「sample」の要素を全て取得するためには、以下のようなPythonコードを書きます。
from bs4 import BeautifulSoup html = """ <html> <head> <title>サンプル</title> </head> <body> <div class="sample">これはサンプルです</div> <div class="sample">これもサンプルです</div> <div class="example">これは例です</div> </body> </html> """ soup = BeautifulSoup(html, "html.parser") div_tags = soup.find_all("div", {"class": "sample"}) for div_tag in div_tags: print(div_tag.string)
実行結果は以下のようになります。
これはサンプルです これもサンプルです
上記のコードでは、BeautifulSoupオブジェクトを作成し、<div>要素のclass属性が「sample」の要素を全て取得しています。そして、forループを使って各要素の文字列を表示しています。
BeautifulSoupで属性値による絞り込み検索方法
次に、BeautifulSoupを使って属性値による絞り込み検索する方法について解説します。以下のようなHTMLがあったとします。
<html> <head> <title>サンプル</title> </head> <body> <div class="sample">これはサンプルです</div> <div class="example">これは例です</div> <div class="sample" data-id="1">これはサンプルです</div> <div class="sample" data-id="2">これもサンプルです</div> </body> </html>
このHTMLの中で、data-id属性が「2」の要素を取得するためには、以下のようなPythonコードを書きます。
from bs4 import BeautifulSoup html = """ <html> <head> <title>サンプル</title> </head> <body> <div class="sample">これはサンプルです</div> <div class="example">これは例です</div> <div class="sample" data-id="1">これはサンプルです</div> <div class="sample" data-id="2">これもサンプルです</div> </body> </html> """ soup = BeautifulSoup(html, "html.parser") div_tag = soup.find("div", {"data-id": "2"}) if div_tag: print(div_tag.string) else: print("該当する要素はありません")
実行結果は以下のようになります。
これもサンプルです
上記のコードでは、BeautifulSoupオブジェクトを作成し、data-id属性が「2」の要素を取得しています。そして、該当する要素が存在する場合にはその文字列を表示し、存在しない場合には「該当する要素はありません」と表示しています。
BeautifulSoupで複数の属性を持つ要素の検索方法
最後に、BeautifulSoupを使って複数の属性を持つ要素を検索する方法について解説します。以下のようなHTMLがあったとします。
<html> <head> <title>サンプル</title> </head> <body> <div class="sample" data-id="1">これはサンプルです</div> <div class="example" data-id="2">これは例です</div> <div class="sample" data-id="3" data-type="a">これはサンプルです</div> <div class="sample" data-id="4" data-type="b">これもサンプルです</div> </body> </html>
このHTMLの中で、class属性が「sample」で、data-type属性が「b」の要素を取得するためには、以下のようなPythonコードを書きます。
from bs4 import BeautifulSoup html = """ <html> <head> <title>サンプル</title> </head> <body> <div class="sample" data-id="1">これはサンプルです</div> <div class="example" data-id="2">これは例です</div> <div class="sample" data-id="3" data-type="a">これはサンプルです</div> <div class="sample" data-id="4" data-type="b">これもサンプルです</div> </body> </html> """ soup = BeautifulSoup(html, "html.parser") div_tags = soup.find_all("div", {"class": "sample", "data-type": "b"}) for div_tag in div_tags: print(div_tag.string)
実行結果は以下のようになります。
これもサンプルです
上記のコードでは、BeautifulSoupオブジェクトを作成し、class属性が「sample」で、data-type属性が「b」の要素を全て取得しています。そして、forループを使って各要素の文字列を表示しています。
BeautifulSoupを用いた属性の有無や属性値による検索の実例
最後に、BeautifulSoupを用いた属性の有無や属性値による検索の実例をいくつか紹介します。
class属性がある要素を取得する
以下のようなHTMLがあったとします。
<html> <head> <title>サンプル</title> </head> <body> <div class="sample">これはサンプルです</div> <p>これは段落です</p> <div>これは<b>太字</b>の<i>イタリック</i>です</div> </body> </html>
このHTMLの中で、class属性がある要素を全て取得するためには、以下のようなPythonコードを書きます。
from bs4 import BeautifulSoup html = """ <html> <head> <title>サンプル</title> </head> <body> <div class="sample">これはサンプルです</div> <p>これは段落です</p> <div>これは<b>太字</b>の<i>イタリック</i>です</div> </body> </html> """ soup = BeautifulSoup(html, "html.parser") elements = soup.select("[class]") for element in elements: print(element)
実行結果は以下のようになります。
<div class="sample">これはサンプルです</div>
上記のコードでは、BeautifulSoupオブジェクトを作成し、class属性がある要素を全て取得しています。そして、forループを使って各要素を表示しています。
属性値による絞り込み検索をする
以下のようなHTMLがあったとします。
<html> <head> <title>サンプル</title> </head> <body> <div class="sample" data-id="1">これはサンプルです</div> <div class="example" data-id="2">これは例です</div> <div class="sample" data-id="3" data-type="a">これはサンプルです</div> <div class="sample" data-id="4" data-type="b">これもサンプルです</div> </body> </html>
このHTMLの中で、data-id属性が「1」の要素を取得するためには、以下のようなPythonコードを書きます。
from bs4 import BeautifulSoup html = """ <html> <head> <title>サンプル</title> </head> <body> <div class="sample" data-id="1">これはサンプルです</div> <div class="example" data-id="2">これは例です</div> <div class="sample" data-id="3" data-type="a">これはサンプルです</div> <div class="sample" data-id="4" data-type="b">これもサンプルです</div> </body> </html> """ soup = BeautifulSoup(html, "html.parser") elements = soup.select("[data-id='1']") for element in elements: print(element)
実行結果は以下のようになります。
<div class="sample" data-id="1">これはサンプルです</div>
上記のコードでは、BeautifulSoupオブジェクトを作成し、data-id属性が「1」の要素を全て取得しています。そして、forループを使って各要素を表示しています。
まとめ
この記事では、BeautifulSoupを使ってHTMLの属性の有無を検索する方法について解説しました。具体的には、以下の内容を説明しました。
- BeautifulSoupとは何か
- BeautifulSoupを使った属性の有無の確認方法
- BeautifulSoupによるHTMLの特定の属性の検索方法
- BeautifulSoupで属性値による絞り込み検索方法
- BeautifulSoupで複数の属性を持つ要素の検索方法
- BeautifulSoupを用いた属性の有無や属性値による検索の実例
以上の内容を理解し、実際に使いこなせるようになれば、HTMLの解析やスクレイピングにおいて非常に便利なツールとなるでしょう。