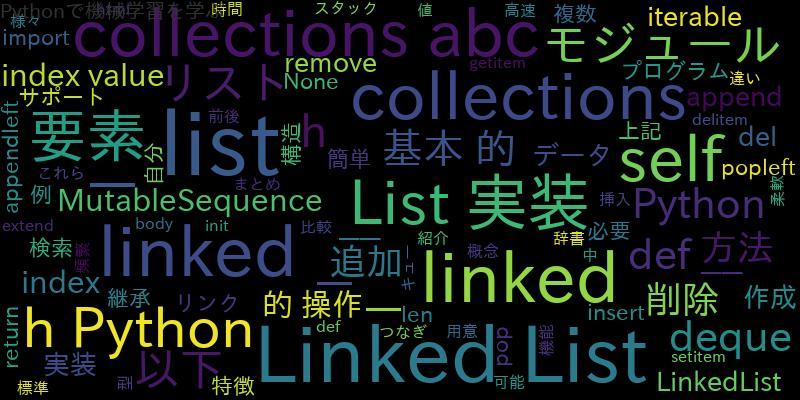
Pythonのcollectionsとcollections.abcモジュールの紹介
Pythonには様々なモジュールが用意されており、その中には「collections」と「collections.abc」があります。これらは、リストや辞書といった標準的なデータ型に機能を追加するためのモジュールです。
Pythonでのリストの基本的な操作
Pythonにおけるリストは、複数の値をまとめたものです。リストは以下のように作成することができます。
my_list = [1, 2, 3, 4, 5] print(my_list)
リストに要素を追加するには、以下のようにします。
my_list.append(6) print(my_list)
リストから要素を削除するには、以下のようにします。
my_list.remove(3) print(my_list)
Linked Listの基本的な概念と特徴
Linked Listは、複数の要素をリンクでつなぎ合わせたデータ構造です。各要素は、前後の要素とのリンクを持っています。
Linked Listの特徴は、要素の挿入や削除が高速に行えることです。ただし、要素の検索には時間がかかるため、データの検索が頻繁に行われる場合には適していません。
Pythonのcollectionsを使った簡易的なLinked Listの実装方法
Pythonのcollectionsモジュールを使って、簡易的なLinked Listを実装することができます。以下は、collectionsモジュールを使ったLinked Listの実装例です。
from collections import deque my_linked_list = deque([1, 2, 3, 4, 5]) print(my_linked_list) my_linked_list.appendleft(0) print(my_linked_list) my_linked_list.pop() print(my_linked_list) my_linked_list.popleft() print(my_linked_list)
上記のプログラムでは、dequeを使ってLinked Listを作成しています。要素の追加には「appendleft」を、要素の削除には「pop」と「popleft」を使っています。
Pythonのcollections.abcを使ったMutableSequenceを継承したカスタムリストの実装例
Pythonのcollections.abcモジュールを使って、MutableSequenceを継承したカスタムリストを実装することもできます。以下は、collections.abcモジュールを使った実装例です。
from collections.abc import MutableSequence class LinkedList(MutableSequence): def __init__(self, iterable=None): self._list = [] if iterable is not None: self.extend(iterable) def __getitem__(self, index): return self._list[index] def __setitem__(self, index, value): self._list[index] = value def __delitem__(self, index): del self._list[index] def __len__(self): return len(self._list) def insert(self, index, value): self._list.insert(index, value) my_linked_list = LinkedList([1, 2, 3, 4, 5]) print(my_linked_list) my_linked_list.append(6) print(my_linked_list) my_linked_list.remove(3) print(my_linked_list) del my_linked_list[0] print(my_linked_list)
上記のプログラムでは、MutableSequenceを継承してLinkedListを作成しています。要素の追加には「append」を、要素の削除には「remove」と「del」を使っています。
まとめ
Pythonのcollectionsを使って、Linked Listを実装する方法について学びました。collectionsは、dequeを使って簡易的なLinked Listを実装することができます。