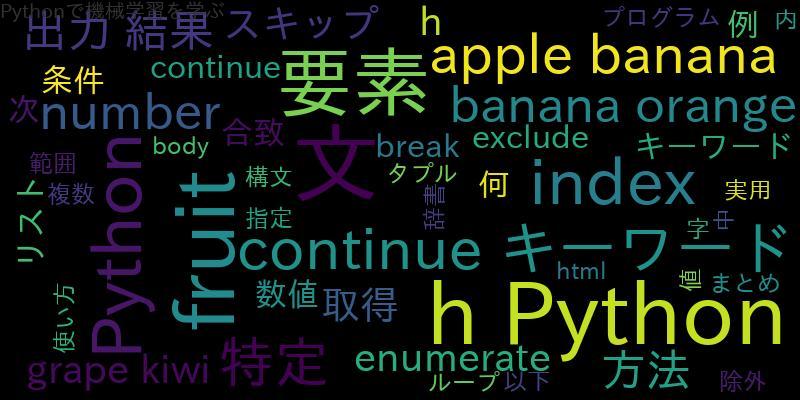
Pythonのfor文とは何か
Pythonのfor文は、指定された範囲内の要素に対して繰り返し処理を行うための構文です。for文を使うことで、リストやタプル、辞書などの複数の要素に対して同じ処理を行うことができます。
Pythonのfor文でindexを取得する方法
Pythonのfor文では、要素の値だけでなく、その要素のindex(添え字)も取得することができます。
fruits = ['apple', 'banana', 'orange'] for i, fruit in enumerate(fruits): print(i, fruit)
出力結果:
0 apple 1 banana 2 orange
Pythonのfor文で特定のindexを抜ける方法
Pythonのfor文で、特定のindexまでの要素のみを処理したい場合は、breakキーワードを使います。
fruits = ['apple', 'banana', 'orange', 'grape', 'kiwi'] for i, fruit in enumerate(fruits): if i == 3: break print(i, fruit)
出力結果:
0 apple 1 banana 2 orange
Pythonのcontinueキーワードとは何か
Pythonのcontinueキーワードは、for文やwhile文の中で使われ、その処理をスキップして、次のループに移るためのキーワードです。
Pythonのfor文でcontinueキーワードを使う方法
Pythonのfor文でcontinueキーワードを使うことで、特定の条件に合致する要素をスキップして、次の要素に進むことができます。
fruits = ['apple', 'banana', 'orange', 'grape', 'kiwi'] for i, fruit in enumerate(fruits): if i == 2: continue print(i, fruit)
出力結果:
0 apple 1 banana 3 grape 4 kiwi
Pythonでfor文とcontinueキーワードを組み合わせた実用例
以下は、数値のリストから特定の数値を除外するプログラムの例です。
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] exclude = [3, 5, 8] for number in numbers: if number in exclude: continue print(number)
出力結果:
1 2 4 6 7 9 10
まとめ
Pythonのfor文では、要素のindexを取得したり、特定のindexまでの要素のみを処理することができます。また、continueキーワードを使うことで、特定の条件に合致する要素をスキップして、処理を続けることができます。