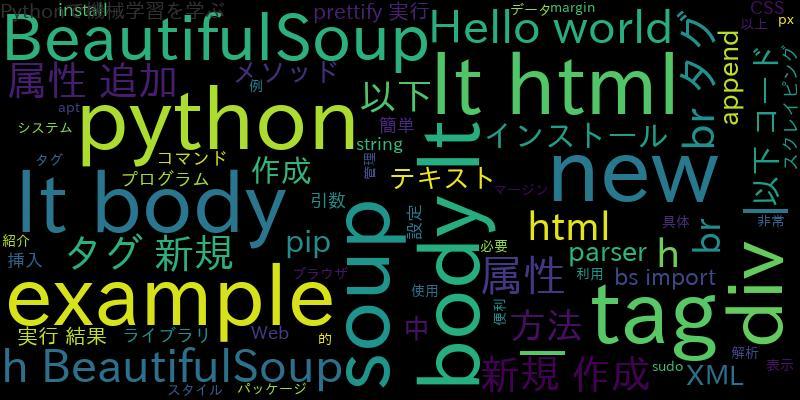
BeautifulSoupは、PythonでHTMLやXMLなどのテキストデータを解析するためのライブラリです。HTMLやXMLのタグや属性を簡単に扱えるため、Webスクレイピングなどのプログラムによく利用されます。
BeautifulSoupのインストール方法
BeautifulSoupを使うには、まずPythonのパッケージ管理システムであるpipを使ってインストールする必要があります。以下のコマンドを実行してください。
pip install beautifulsoup4
pipがインストールされていない場合は、以下のコマンドでインストールしてください。
sudo apt-get install python-pip
BeautifulSoupでの新規タグの作成方法
BeautifulSoupでは、new_tagメソッドを使って新しいタグを作成することができます。以下のコードは、h1タグを新規作成して、その中に「Hello, world!」というテキストを挿入しています。
from bs4 import BeautifulSoup soup = BeautifulSoup("<html><body></body></html>", "html.parser") new_tag = soup.new_tag("h1") new_tag.string = "Hello, world!" soup.body.append(new_tag) print(soup.prettify())
実行結果は以下のようになります。
<html> <body> <h1> Hello, world! </h1> </body> </html>
BeautifulSoupでのclass属性を持つタグの作成方法
class属性を持つタグを作成するには、new_tagメソッドの引数にclass_属性を追加します。以下のコードは、divタグを新規作成して、その中に「Hello, world!」というテキストを挿入し、class属性を追加しています。
from bs4 import BeautifulSoup soup = BeautifulSoup("<html><body></body></html>", "html.parser") new_tag = soup.new_tag("div", class_="example-class") new_tag.string = "Hello, world!" soup.body.append(new_tag) print(soup.prettify())
実行結果は以下のようになります。
<html> <body> <div class="example-class"> Hello, world! </div> </body> </html>
BeautifulSoupでのbrタグの新規作成とclass属性の追加方法
brタグを新規作成して、class属性を追加するには、new_tagメソッドの引数に”br”とclass_属性を追加します。以下のコードは、brタグを新規作成して、class属性を追加しています。
from bs4 import BeautifulSoup soup = BeautifulSoup("<html><body></body></html>", "html.parser") new_tag = soup.new_tag("br", class_="example-class") soup.body.append(new_tag) print(soup.prettify())
実行結果は以下のようになります。
<html> <body> <br class="example-class"/> </body> </html>